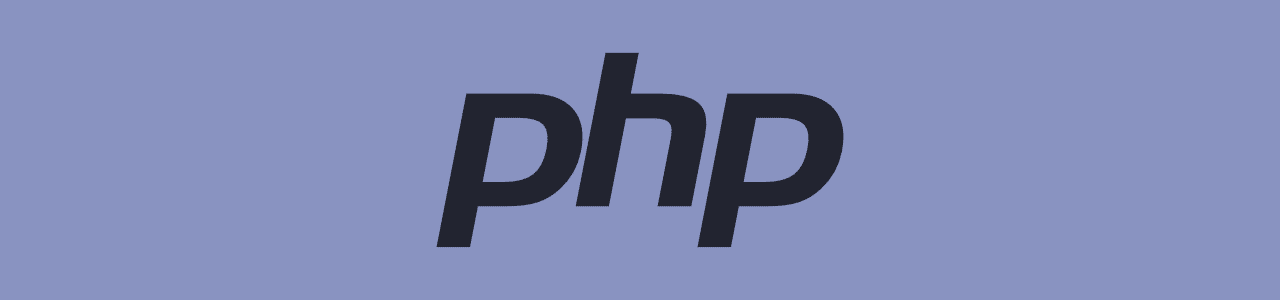
Php Documentation
If you want to show the payment options from our end, you need to follow this documentation. This will redirect you to the payment gateway options.
$post_data = array();
$post_data['clientId'] = "your_client_id";
$post_data['amount'] = "enter_amount_here";
$post_data['uniqueId'] = "unique_id"; //it can be your unique invoice_id
$endpoint = "http://202.74.240.167:8083/payment.php";
$handle = curl_init();
curl_setopt($handle, CURLOPT_URL, $endpoint );
curl_setopt($handle, CURLOPT_TIMEOUT, 30);
curl_setopt($handle, CURLOPT_CONNECTTIMEOUT, 30);
curl_setopt($handle, CURLOPT_POST, 1 );
curl_setopt($handle, CURLOPT_POSTFIELDS, $post_data);
curl_setopt($handle, CURLOPT_RETURNTRANSFER, true);
curl_setopt($handle, CURLOPT_SSL_VERIFYPEER, FALSE); //false for local environment
$content = curl_exec($handle);
$code = curl_getinfo($handle, CURLINFO_HTTP_CODE);
if($code == 200 && !( curl_errno($handle))) {
curl_close( $handle);
$response = $content;
} else {
curl_close($handle);
echo "Failed to connect with API";
exit;
}
$parse = json_decode($response,true);
if (isset($parse['gateURL'])) {
header("Location: ".$parse['gateURL']);
} else {
echo "Data parsing error!";
}